
With the advancement of web development in the world we live in, React, a javascript library, emerges as an attraction for both experienced and beginners. But what are React Hooks? In simpler form, these are built-in functions that enable us to hook into React state and lifecycle features from functional (stateless) components.
With hooks, you can manage state, and other React features without re-rendering functional components into class components. This let you use a variety of react features from your component. This blog explores the key aspects of the react hooks, including what is the purpose of the hook. By the end of this comprehensive blog, we take you through the custom react hook js, react js fundamentals and call backs to make your life easier. With that in mind, let’s get started!
What is React-hook form?
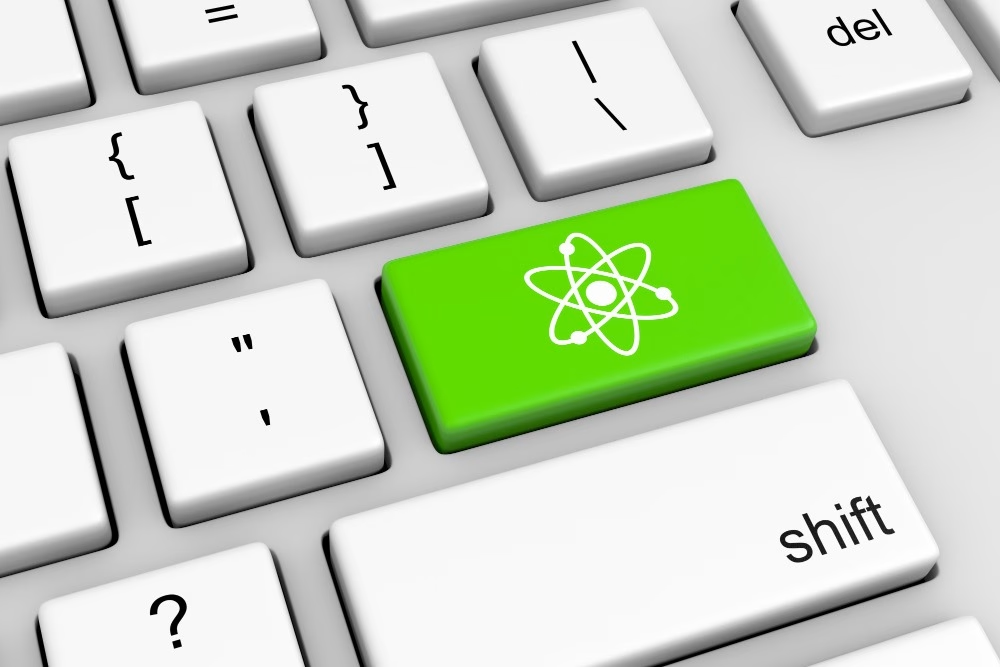
Before we go into the details of the react hook and its aspects in details, lets first talk about what is react hook form is. This means a library that can help manage complex forms. As you continue further into the different react hook tutorials or self-study, you should be aware that hooks cannot be used inside class components; they are exclusive to functional components.
React Hooks not only allow state management but also provide an alternative to various lifecycle methods such as componentDidMount
, componentDidUpdate
, and componentWillUnmount
. Rather you can take advantage of useEffect
, a built-in hook, to handle these lifecycle events.
Why Use React Hooks?
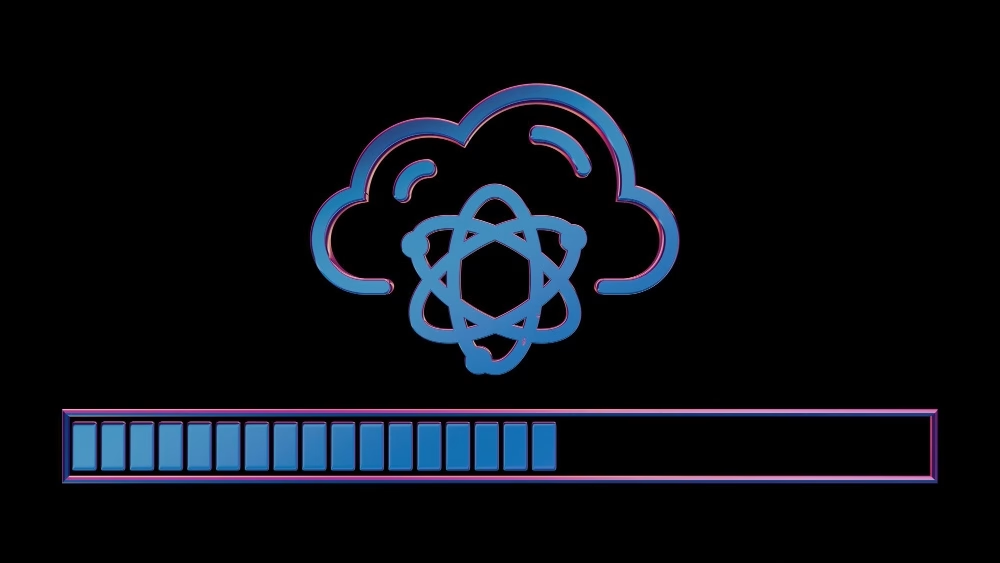
Functional components in React are known for their simplicity and benefits. However, managing stateful logic across components has traditionally been challenging. Techniques like render props and higher-order components have been used to share reusable behaviors, but they often result in complex and difficult-to-understand code structures, sometimes referred to as “wrapper hell.”
React Hooks address these issues by allowing you to reuse stateful logic without altering your component hierarchy. This results in cleaner, more understandable code. Hooks also help in breaking down a component into smaller, more manageable functions, reducing bugs and inconsistencies that arise from intertwined lifecycle methods.
What are the best React Hooks?
React hook form embraces native HTML form validation.
- useState: useState manages state within a functional component.
- useEffect: useEffect handles side effects and lifecycle events within a functional component.
- useContext: useContext retrives the current context value provided by the nearest context provider.
These are some of the main types of ReactHooks, that programmers find it quite beneficial as they result into the out of the box integration to each UI library and manage lifecycle events. Regardlgess, useState
is particularly significant for state management in functional components. After knowing the types, lets shift our focus into how useState
works.
Read More: SQL Queries for practice
UseState Hook
useState is a fundamental hook that enables you to add state to functional components. It allows you to initialize the state and provides a function to update that state. The example that shows the proper use of useState Hook to enhance the complex library is as follows.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, useState
initializes count
to 0
and provides a function setCount
to update it. Each time the button is clicked, setCount
increments the count
by one.
Why Use UseContext?
The useContext is used to consume values from the React Context. It provides a way to pass the data through the tree components without having to pass the props manually at every level.
React Context to Avoid Prop-Drilling
React’s Context API provides a way to pass data through the component tree without having to manually pass props at every level. Here’s a simple example to illustrate its usage.
First, we create a Context object using the createContext()
function:
javascriptCopy codeimport React, { createContext } from "react";
const Context = createContext();
To make the context available throughout the component tree, we wrap the parent component with the Context provider. In this case, our parent component is <App />
:
javascriptCopy codeimport React, { createContext } from "react";
const Context = createContext();
const App = () => {
return <Context.Provider>/* ... */</Context.Provider>;
};
Next, we specify the context value using the value
prop of <Context.Provider>
. This value will be accessible by any component within the tree:
javascriptCopy codeimport React, { createContext } from "react";
const Context = createContext();
const App = () => {
return (
<Context.Provider value={{ data: "Data from context!" }}>
{/* ... */}
</Context.Provider>
);
};
Suppose <App />
renders a child component named <Child />
:
javascriptCopy codeimport React, { createContext } from "react";
const Context = createContext();
const Child = () => {
return <div>This is the child component!</div>;
};
const App = () => {
return (
<Context.Provider value={{ data: "Data from context!" }}>
<Child />
</Context.Provider>
);
};
To access the context value within the <Child />
component, we use the useContext
hook:
javascriptCopy codeimport React, { createContext, useContext } from "react";
const Context = createContext();
const Child = () => {
const context = useContext(Context);
return <div>{context.data}</div>;
};
const App = () => {
return (
<Context.Provider value={{ data: "Data from context!" }}>
<Child />
</Context.Provider>
);
};
With these changes, the data from the parent <App />
component is rendered in the <Child />
component using the Context API.
Example with Multiple Nested Components
Context’s benefits become more apparent when dealing with deeply nested components. Let’s assume we have multiple child components nested within each other:
javascriptCopy codeimport React, { createContext, useContext } from "react";
const Context = createContext();
const Child5 = () => {
const context = useContext(Context);
return <div>{context.data}</div>;
};
const Child4 = () => {
return <Child5 />;
};
const Child3 = () => {
return <Child4 />;
};
const Child2 = () => {
return <Child3 />;
};
const Child = () => {
return <Child2 />;
};
const App = () => {
return (
<Context.Provider value={{ data: "Data from context!" }}>
<Child />
</Context.Provider>
);
};
In this example, the <Child5 />
component, which is the deepest in the hierarchy, accesses and renders the context data value. This demonstrates how Context eliminates the need for prop-drilling, as the context data is available to any component within the provider’s tree.
By using Context, data from the parent <App />
component is seamlessly accessed in the <Child5 />
component, showcasing the power and convenience of React’s Context API.
Conclusion
React Hooks, especially useState
, simplify state management and enhance the modularity and readability of your React components. By using hooks, you can maintain the functional component paradigm while effectively managing state and lifecycle events.
In this blog, we discussed the features of React Hooks, react hook forms and its essentials. We hope you find this helpful. If you seek further insights into various aspects of React, look no further than Prog Culers. Thank you for reading till the end!